Two Best Solutions :
function create_instance($class, $params) {
return call_user_func_array(
array(new ReflectionClass($class), 'newInstance'),
$params
);
}
or
function create_instance($class, $params) {
$reflection_class = new ReflectionClass($class);
return $reflection_class->newInstanceArgs($params);
}
Please follow this blog if u feel the blog content is useful to you
Thursday, September 22, 2011
Custom Theme the maintenance ("Site Offline") page
Step 1: Override the maintenance page template
Copy your main page.tpl.php and rename it to maintenance-page.tpl.php or copy the template located in modules/system/maintenance-page.tpl.php to your theme
Step 2: Indicate in your settings.php file
specify the theme name in which u have put the maintenance-page.tpl.php in step 1
this has to be in settings.php
<?php
$conf['maintenance_theme'] = 'themeName';
?>
Step 3: Create the maintenance offline page in the case of database failure
event warnings about the database connection from appearing, copy your page.tpl.php file and rename it maintenance-page-offline.tpl.php. Change the $content variable with your custom message. Note, this template file is a template suggestion based on maintenance-page.tpl.php so they both need to exist in the same folder.
At the top of maintenance-page-offline.tpl.php, you might also want to set the following variables detailed in step 4.
Step 4: Hard code site settings
In this mode, your database is inaccessible, therefore some key settings about your site are not available. If you want this page to use the same theme and other settings that the site would normally use, you must replicate those settings by using hard-coded values.
<?php
$head_title = 'mysite.com :: Site-offline';
$logo = 'sites/all/files/customLogo.png';
// If your theme is set to display the site name, uncomment this line and replace the value:
// $site_name = 'Your Site Name';
// If your theme is set to *not* display the site name, uncomment this line:
unset($site_name);
// If your theme is set to display the site slogan, uncomment this line and replace the value:
$site_slogan = 'My Site Slogan';
// If your theme is set to *not* display the site slogan, uncomment this line:
// unset($site_slogan);
// Main message. Note HTML markup.
$content = 'The site is currently not available due to technical problems. Please try again later. Thank you for your understanding.If you are the maintainer of this site, please check your database settings.';
?>
Copy your main page.tpl.php and rename it to maintenance-page.tpl.php or copy the template located in modules/system/maintenance-page.tpl.php to your theme
Step 2: Indicate in your settings.php file
specify the theme name in which u have put the maintenance-page.tpl.php in step 1
this has to be in settings.php
<?php
$conf['maintenance_theme'] = 'themeName';
?>
Step 3: Create the maintenance offline page in the case of database failure
event warnings about the database connection from appearing, copy your page.tpl.php file and rename it maintenance-page-offline.tpl.php. Change the $content variable with your custom message. Note, this template file is a template suggestion based on maintenance-page.tpl.php so they both need to exist in the same folder.
At the top of maintenance-page-offline.tpl.php, you might also want to set the following variables detailed in step 4.
Step 4: Hard code site settings
In this mode, your database is inaccessible, therefore some key settings about your site are not available. If you want this page to use the same theme and other settings that the site would normally use, you must replicate those settings by using hard-coded values.
<?php
$head_title = 'mysite.com :: Site-offline';
$logo = 'sites/all/files/customLogo.png';
// If your theme is set to display the site name, uncomment this line and replace the value:
// $site_name = 'Your Site Name';
// If your theme is set to *not* display the site name, uncomment this line:
unset($site_name);
// If your theme is set to display the site slogan, uncomment this line and replace the value:
$site_slogan = 'My Site Slogan';
// If your theme is set to *not* display the site slogan, uncomment this line:
// unset($site_slogan);
// Main message. Note HTML markup.
$content = 'The site is currently not available due to technical problems. Please try again later. Thank you for your understanding.If you are the maintainer of this site, please check your database settings.';
?>
Overriding other theme functions
If you want to override a theme function not included in the basic list (block, box, comment, node, page), you need to tell PHPTemplate about it.
To do this, you need to create a template.php file in your theme's directory. This file must start with a PHP opening tag <?php but the close tag is not needed and it is recommended that you omit it. Also included in the file are stubs for the theme overrides. These stubs instruct the engine what template file to use and which variables to pass to it.
First, you need to locate the appropriate theme function to override. You can find a list of these in the API documentation. We will use theme_item_list() as an example.
The function definition for theme_item_list() looks like this:
<?php
function theme_item_list($items = array(), $title = NULL) {
$output = '<div class="item-list">';
if (isset($title)) {
$output .= '<h3>'. $title .'</h3>';
}
?>
Now you need to place a stub in your theme's template.php, like this:
<?php
/**
* Catch the theme_item_list function, and redirect through the template api
*/
function phptemplate_item_list($items = array(), $title = NULL) {
// Pass to phptemplate, including translating the parameters to an associative array.
// The element names are the names that the variables
// will be assigned within your template.
return _phptemplate_callback('item_list', array('items' => $items, 'title' => $title));
}
?>
We replaced the word theme in the function name with phptemplate and used a call to _phptemplate_callback() to pass the parameters ($items and $title) to PHPTemplate.
Now, you can create a file item_list.tpl.php file in your theme's directory, which will be used to theme item lists. Start by putting in the code from the original theme_item_list(). Do not wrap this code into a function, write inline, e.g.
<?php
$output = '<div class="item-list">';
if (isset($title)) {
$output .= '<h3>'. $title .'</h3>';
}
?>
To do this, you need to create a template.php file in your theme's directory. This file must start with a PHP opening tag <?php but the close tag is not needed and it is recommended that you omit it. Also included in the file are stubs for the theme overrides. These stubs instruct the engine what template file to use and which variables to pass to it.
First, you need to locate the appropriate theme function to override. You can find a list of these in the API documentation. We will use theme_item_list() as an example.
The function definition for theme_item_list() looks like this:
<?php
function theme_item_list($items = array(), $title = NULL) {
$output = '<div class="item-list">';
if (isset($title)) {
$output .= '<h3>'. $title .'</h3>';
}
?>
Now you need to place a stub in your theme's template.php, like this:
<?php
/**
* Catch the theme_item_list function, and redirect through the template api
*/
function phptemplate_item_list($items = array(), $title = NULL) {
// Pass to phptemplate, including translating the parameters to an associative array.
// The element names are the names that the variables
// will be assigned within your template.
return _phptemplate_callback('item_list', array('items' => $items, 'title' => $title));
}
?>
We replaced the word theme in the function name with phptemplate and used a call to _phptemplate_callback() to pass the parameters ($items and $title) to PHPTemplate.
Now, you can create a file item_list.tpl.php file in your theme's directory, which will be used to theme item lists. Start by putting in the code from the original theme_item_list(). Do not wrap this code into a function, write inline, e.g.
<?php
$output = '<div class="item-list">';
if (isset($title)) {
$output .= '<h3>'. $title .'</h3>';
}
?>
Making additional variables available to your templates
Example 1:
<?php
function _phptemplate_variables($hook, $vars) {
switch($hook) {
case 'comment' :
$vars['newvar'] = 'new variable';
$vars['title'] = 'new title';
break;
}
return $vars;
}
?>
Example 2:
<?php
function _phptemplate_variables($hook, $vars) {
switch ($hook) {
case 'page':
if (arg(0) == 'node' && is_numeric(arg(1)) && arg(2) == '') {
$vars['content_is_node'] = TRUE;
}
break;
}
return $vars;
}
?>
<?php
function _phptemplate_variables($hook, $vars) {
switch($hook) {
case 'comment' :
$vars['newvar'] = 'new variable';
$vars['title'] = 'new title';
break;
}
return $vars;
}
?>
Example 2:
<?php
function _phptemplate_variables($hook, $vars) {
switch ($hook) {
case 'page':
if (arg(0) == 'node' && is_numeric(arg(1)) && arg(2) == '') {
$vars['content_is_node'] = TRUE;
}
break;
}
return $vars;
}
?>
Theming blocks individually, by region, or by module
Designers can create multiple tpl.php files for blocks based on the specific block, the module that created the block, or the region that the block appears in.
Template files are searched in the following order:
block-[module]-[delta].tpl.php
block-[module].tpl.php
block-[region].tpl.php
block.tpl.php
For example, the user login block has a delta of '0'. If you put it in the left sidebar, when it's rendered, PHPTemplate will search for the following templates in descending order:
block-user-0.tpl.php
block-user.tpl.php
block-left.tpl.php
block.tpl.php
You can find the block's module and delta by looking at the html source of a page: each block's main DIV has the following classes and IDs:
<div class="block block-{module}" id="block-{module}-{delta}">
Note that custom blocks created in the admin UI count as coming block.module, so their template is 'block-block-[delta]'.
Template files are searched in the following order:
block-[module]-[delta].tpl.php
block-[module].tpl.php
block-[region].tpl.php
block.tpl.php
For example, the user login block has a delta of '0'. If you put it in the left sidebar, when it's rendered, PHPTemplate will search for the following templates in descending order:
block-user-0.tpl.php
block-user.tpl.php
block-left.tpl.php
block.tpl.php
You can find the block's module and delta by looking at the html source of a page: each block's main DIV has the following classes and IDs:
<div class="block block-{module}" id="block-{module}-{delta}">
Note that custom blocks created in the admin UI count as coming block.module, so their template is 'block-block-[delta]'.
Import & export database in mysql using command line
MysqlExport :
Go to bin directory
mysqldump –u expuser –p dbname --routines > c:/file.sql
Press enter
Enter Password:
Enter your password
You are done with it
MysqlImport :
Go to bin directory
mysql –uexpuser –p dbname < c:/dile.sql
Press Enter
Enter Password: will appear
Enter your password
You are done with it
Another way is:
Connect with mysql in commond prompt
mysql>Create database dbname;
mysql>User dbname;
mysql>Source file.sql; ExpertusONE_db.sql
Go to bin directory
mysqldump –u expuser –p dbname --routines > c:/file.sql
Press enter
Enter Password:
Enter your password
You are done with it
MysqlImport :
Go to bin directory
mysql –uexpuser –p dbname < c:/dile.sql
Press Enter
Enter Password: will appear
Enter your password
You are done with it
Another way is:
Connect with mysql in commond prompt
mysql>Create database dbname;
mysql>User dbname;
mysql>Source file.sql; ExpertusONE_db.sql
Adding Language Icons in drupal
In Drupal, language flags can be easily included in the language switcher with the Language Icons modulehttp://drupal.org/project/languageicons.
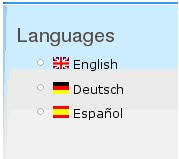
Go to http://drupal.org/project/languageicons and download the module and install in your sites/all/modules directory.
Navigate to the module admisistration page in your website, /admin/build/modules, and enable the "Language Icons" module listed under the "Multilanguage" section.
Then save the configuration and that's it, you should now have flags in your language switcher.
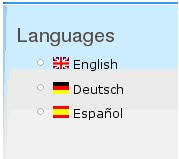
Go to http://drupal.org/project/languageicons and download the module and install in your sites/all/modules directory.
Navigate to the module admisistration page in your website, /admin/build/modules, and enable the "Language Icons" module listed under the "Multilanguage" section.
Then save the configuration and that's it, you should now have flags in your language switcher.
Subscribe to:
Posts (Atom)